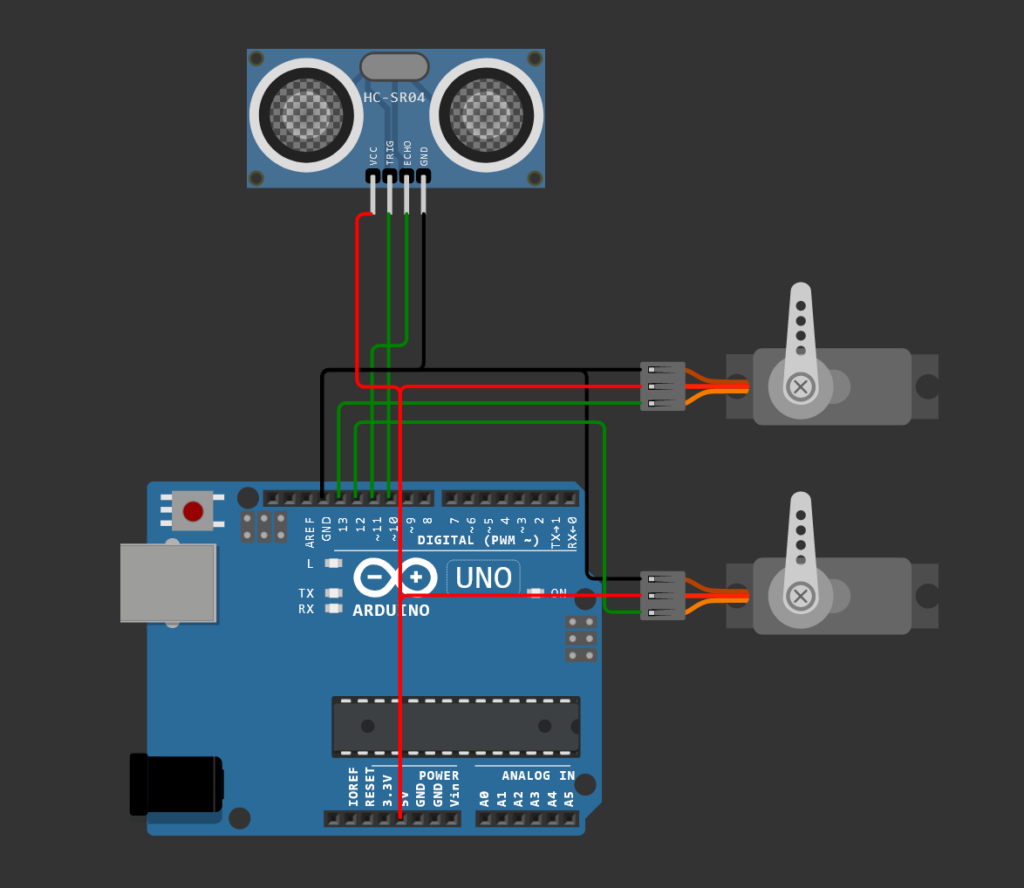
When learning Arduino in my freshman year, I had the chance to do our own projects. I was looking for unique ideas, and focused on the ultrasonic sensor. This sensor can provide a distance value for a point.
What if these dots were arranged into a line, then those lines were arranged into a plane? What if each data point was given a color so it could be more visual? It would be like taking a picture with a camera, albeit a lot slower.
Circuit
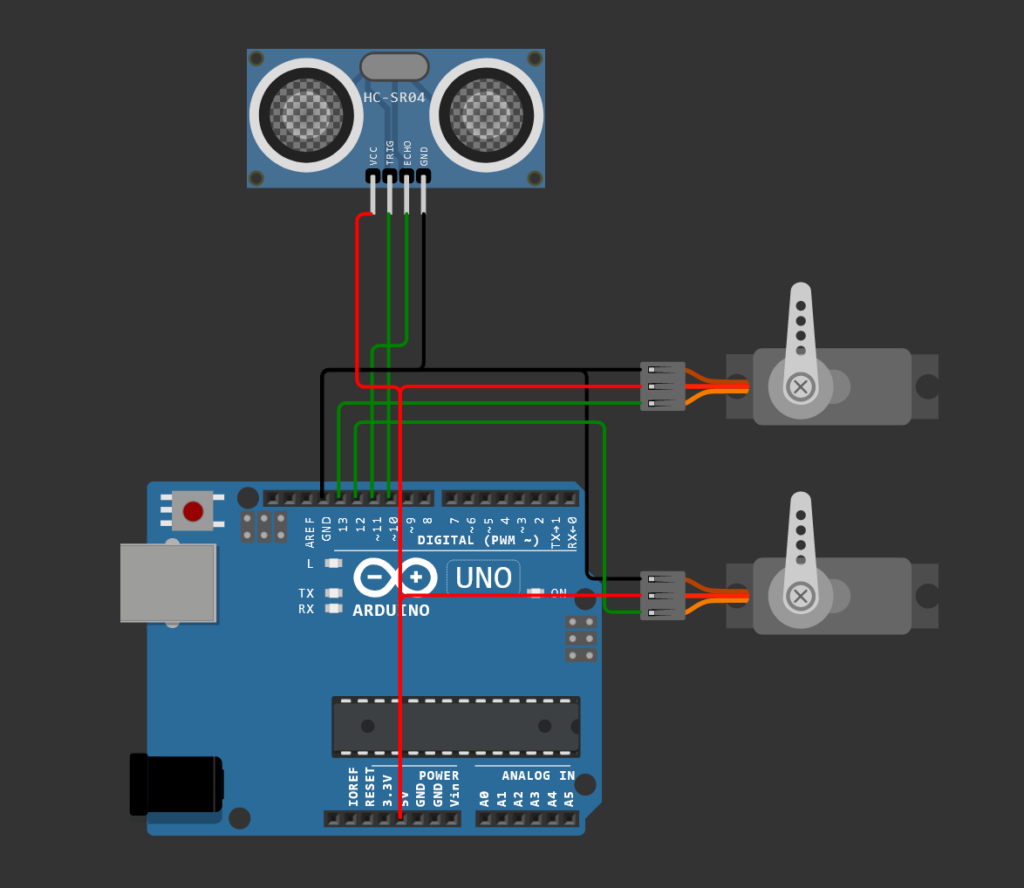
The circuit is very simple. The bottom servo motor will control the up and down tilt of the ultrasonic sensor, and the top servo will swivel the sensor left and right.
Code
#include <Servo.h>
// Pins for the ultrasonic sensor
const int trigPin = 10;
const int echoPin = 11;
long duration;
int distance;
Servo servo_x; // Servo for swivel left/right
Servo servo_y; // Servo for swivel up/down
void setup() {
// Set up sensor pins, serial, and servo pins
pinMode(trigPin, OUTPUT);
pinMode(echoPin, INPUT);
Serial.begin(9600);
servo_x.attach(12);
servo_y.attach(13);
}
void loop() {
// Sweep up to down
for(int i = 105; i > 60; i = i - 3) {
servo_y.write(i);
// Sweep left to right
for(int j = 15; j <= 165; j++) {
servo_x.write(j);
delay(30);
distance = calculateDistance();
// Clamp max distance to 255
if(distance > 255) {
distance = 255;
}
// Print serial data using format:
// "horizontalAngle/verticalAngle,distance."
Serial.print(j);
Serial.print("/");
Serial.print(i);
Serial.print(",");
Serial.print(distance);
Serial.print(".");
}
}
}
// Apply formula to derive distance
int calculateDistance() {
digitalWrite(trigPin, LOW);
delayMicroseconds(2);
digitalWrite(trigPin, HIGH);
delayMicroseconds(10);
digitalWrite(trigPin, LOW);
duration = pulseIn(echoPin, HIGH);
distance = duration * 0.034 / 2;
return distance;
}
Running the code, the sensor sweeps left to right, measuring distances every time the servo moves one degree.
Completing one line, the bottom servo shifts down three degrees and begins a new scan line.
If all lines of the picture is complete, the sensor will go back to the initial position and update the pixels.
Since the serial comms will be printing the data, there will have to be a way to visualize this.